The Iterator Pattern
— software development, coding, pluralsight, design patterns — 1 min read
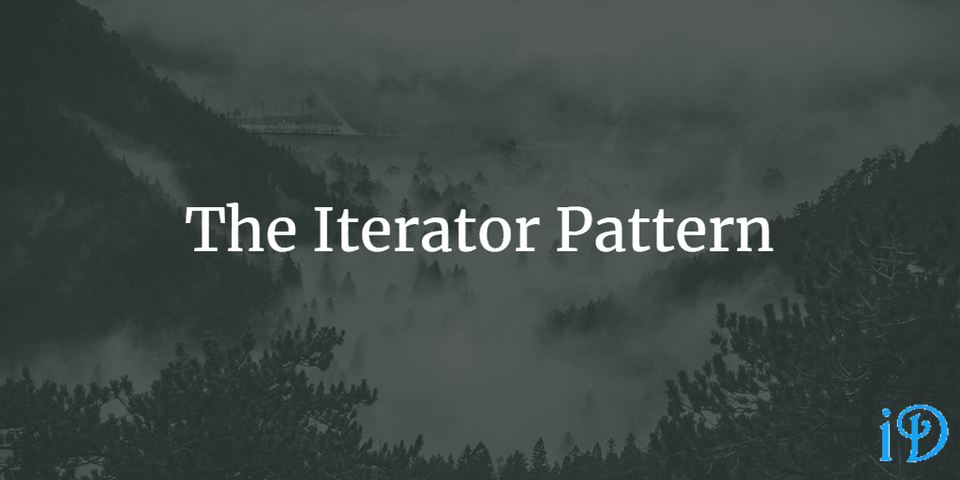
The goal of the Iterator Pattern is to provide an interface which allows the client to iterate through an aggregate object without having to know the implementation of the object. You may also know iterators as cursors.
Head First Design Patterns by Eric Freeman and Elisabeth Freeman gives a pretty good overview of the Iterator Pattern in its ninth chapter.
Pluralsight's Design Patterns Library course has a module on the Iterator Pattern from Steve Smith.
Information hiding and encapsulation of change are pretty essential characteristics of good software. These can be tricky to adhere to, though, if your program is working with several different types of aggregate objects. The Iterator Pattern can help in this scenario.
The goal of the Iterator Pattern is to hide the implementation of each aggregate object behind one consistent interface.
To achieve this, concrete iterators implement an iterator interface, which requires methods hasNext()
and next()
, and sometimes others.
Each aggregate that implements the iterator pattern must implement an aggregator interface. This interface ensures that the aggregate is responsible for creating its own instance of a concrete iterator, which the client can then call upon when it wants to iterate through the aggregate object.
C# actually provides some implementation of this pattern through the IEnumerable
(aggregate) and IEnumerator
(iterator) interfaces. C#'s foreach feature functions on any type that implements IEnumerable
. Other languages, like Java, also have built-in implementations of the Iterator Pattern.
The Iterator Pattern can be used pretty effectively in combination with the Factory Pattern; a factory method is often used in the aggregate to construct iterators.
The Iterator Pattern is also related to the Composite Pattern.
Thanks for reading! I hope you find this and other articles here at ilyanaDev helpful! Be sure to follow me on Twitter @ilyanaDev.